2022. 5. 3. 00:00ㆍASPNET/Blazor
구문 분석할 수 없는 값
사용자가 데이터 바인딩된 요소에 구문 분석할 수 없는 값을 제공하면 바인딩 이벤트가 트리거될 때 구문 분석할 수 없는 값이 자동으로 이전 값으로 되돌아간다.
Pages/UnparsableValues.razor
@page "/unparseable-values"
<p>
<input @bind="inputValue" />
</p>
<p>
<code>inputValue</code>: @inputValue
</p>
@code {
private int inputValue = 123;
}
위 내용에서 다음과 같이 @bind:event="oninput" 으로 변경 하시면 input 에 아주 간단하게
int 를 제외하고는 입력할 수 없게 만들 수 있다.
변경된 아래에 예제를 보자
@page "/unparseable-values"
<p>
<input @bind="inputValue" @bind:event="oninput" /> // <--- @bind:event="oninput" 이 추가됨
</p>
<p>
<code>inputValue</code>: @inputValue
</p>
@code {
private int inputValue = 123;
}
이와 같이 변경 후 실행 하고 int 이외의 값들을 입력해 보자.
입력함과 동시에 각 값들이 사라지는 것을 알 수 있다.
간단한 validation 은 이것을 통해 처리 하자 (일일이 빨간글로 표시할 필요는 없다)
형식 문자열
@bind:format="{FORMAT STRING}" 을 통하여 DateTime 형식 문자열을 표현할 수 있다.
현재 통화 숫자 형식과 같은 다른 형식은 사용할 수 없지만 향 후 릴리스에서 추가될 수 있다.
Pages/DateBinding.razor
@page "/date-binding"
<p>
<label>
<code>yyyy-MM-dd</code> format:
<input @bind="startDate" @bind:format="yyyy-MM-dd" />
</label>
</p>
<p>
<code>startDate</code>: @startDate
</p>
@code {
private DateTime startDate = new(2020, 1, 1);
}
사용자 지정 바인딩 형식
소수 자릿수가 최대 3자리인 양의 소수 또는 음의 소수를 input 에 바인딩 하는 예제
@page "/decimal-binding"
@using System.Globalization
<p>
<label>
Decimal value (±0.000 format):
<input @bind="DecimalValue" />
</label>
</p>
<p>
<code>decimalValue</code>: @decimalValue
</p>
@code {
private decimal decimalValue = 1.1M;
private NumberStyles style =
NumberStyles.AllowDecimalPoint | NumberStyles.AllowLeadingSign;
private CultureInfo culture = CultureInfo.CreateSpecificCulture("en-US");
private string DecimalValue
{
get => decimalValue.ToString("0.000", culture);
set
{
if (Decimal.TryParse(value, style, culture, out var number))
{
decimalValue = Math.Round(number, 3);
}
}
}
}
매개 변수를 사용하여 바인딩 (parameter binding)
Parent 의 Html element 와 Child 의 Property ([Parameter]) 를 연결할 경우
@bind-{Property} 구문을 이용하고 {Property} 는 Child 의 Parameter 가 된다.
Child 에서는 Parent 로 bind 구문을 통해 바이딩 할 수 없다.
그래서 EventCallBack 을 이용한다.
아래 예제 코드를 확인 하자
Pages/Child.razor
<div class="card bg-light mt-3" style="width:18rem ">
<div class="card-body">
<h3 class="card-title">Child Component</h3>
<p class="card-text">
Child <code>Year</code>: @Year
</p>
<button @onclick="UpdateYearFromChild">Update Year from Child</button>
</div>
</div>
@code {
private Random r = new();
[Parameter]
public int Year { get; set; }
[Parameter]
public int Day { get; set; }
[Parameter]
public EventCallback<int> YearChanged { get; set; }
private async Task UpdateYearFromChild()
{
await YearChanged.InvokeAsync(r.Next(1950, 2021));
}
}
Pages/Parent.razor
@page "/Parent"
<h1>Parent Component</h1>
<p>Parent <code>year</code>: @year</p>
<button @onclick="UpdateYear">Update Parent <code>year</code></button>
<Child @bind-Year="year"/>
@code {
private Random r = new();
private int year = 1979;
private void UpdateYear()
{
year = r.Next(1950, 2021);
}
}
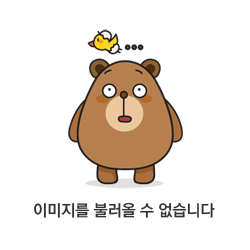
여기서 c# 코드를 아시는 분들은 약간의 의문점이 생길 수 있다.
parent 에서 child 로의 전달 메커니즘은 이해 하겠는데
반대로 child 로 부터 parent 로의 전달 메커니즘은 어떻게 되는 건지 도통 알 수가 없다.
MS($) 가 너무 개발자들을 위해서(??) code 를 숨겨서 생성하는 경향이 있어서 그렇다.
Parent 의 child @bind 코드를 보자
...
<Child @bind-Year="year"/>
...
위의 코드는 아래와 같은 구분이 숨겨진 형태 이다.
...
<Child @bind-Year="year" @bind-Year:event="YearChanged" />
...
즉 YearChanged 라는 handler 를 event 를 통해 year 변수와 연결하기 때문에
child 로 부터 update 시에 parent 의 year 도 변경되게 된다.
확인하고 싶다면 child 의 YearChanged 의 이름을 바꾸면 Parent 로딩시에 에러가 날것이다.
관련영상
'ASPNET > Blazor' 카테고리의 다른 글
이벤트 처리 (0) | 2022.05.05 |
---|---|
Binding 3 (0) | 2022.05.04 |
Binding 1 (0) | 2022.05.02 |
DynamicComponent (0) | 2022.04.29 |
JavaScript Interop - CallingCSharpMethod (0) | 2022.04.28 |