ASPNET 6 Web Api Basic Tutorial 1 / 2 (Swagger, SeriLog, MediatR, EntityFrameworkCore, Scrutor)
2022. 1. 1. 23:52ㆍASPNET/WebApi
반응형
작성 순서
- Create Project
- Create Controller
- Add Swagger
- Add SeriLog
- Add MediatR
- Add EntityFramework Core
- Add Migration
- Apply Database
- Add Web Api
- Refactoring with Extension Method
1. Create Project
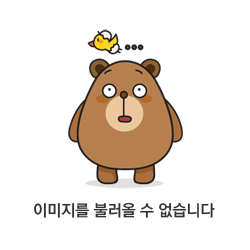
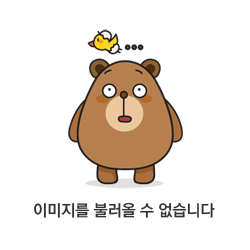
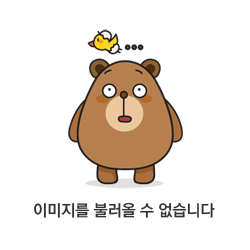
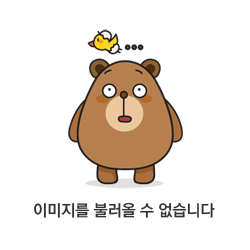
Create
2. Create Controller
Add NewFolder to project (Controller)
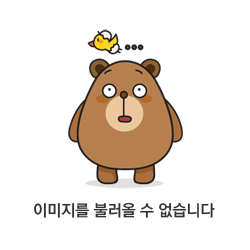
using Microsoft.AspNetCore.Mvc;
namespace WebApiBasicTutorial.Controller
{
[ApiController]
[Route("[controller]")]
public class UserController:ControllerBase
{
}
}
Program.cs 에 다음 코드 추가
삭제 ==> app.MapGet("/", () => "Hello World!");
추가 ==> builder.Services.AddControllers();
3. Add Swagger
개발자 프롬프트에서 프로젝트 폴더로 이동
(현재 .sln 파일이 있는 폴더라면 .csproj 파일이 있는 폴더로 이동)
아래 명령 실행
dotnet add package Swashbuckle.AspNetCore
Program.cs 에 다음 코드 추가
builder.Services.AddControllers();
builder.Services.AddEndpointsApiExplorer();
app.UseSwagger();
app.UseSwaggerUI();
Properties --> launchSettings.json --> "launchUrl": "swagger", 추가
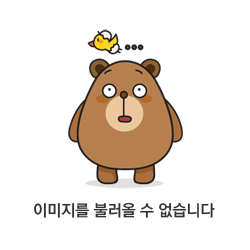
4. Add SeriLog
개발자 프롬프트에서 프로젝트 폴더로 이동
(현재 .sln 파일이 있는 폴더라면 .csproj 파일이 있는 폴더로 이동)
아래 명령 실행
dotnet add package Serilog.AspNetCore
dotnet add package Serilog.Expressions
dotnet add package Serilog.Sinks.Seq
Program.cs 에 다음 코드 추가
using Serilog;
...
var configuration = new ConfigurationBuilder()
.AddJsonFile("appsettings.json", false, true)
.AddJsonFile($"appsettings.Development.json", optional: true)
.Build();
Log.Logger = new LoggerConfiguration()
.ReadFrom.Configuration(configuration)
.CreateBootstrapLogger();
builder.Host.UseSerilog((ctx, lc) => lc
.ReadFrom.Configuration(ctx.Configuration));
appsettings.json 에 다음 추가
"Serilog": {
"MinimumLevel": {
"Default": "Information",
"Override": {
"Microsoft": "Warning",
"Microsoft.Hosting.Lifetime": "Information",
"System": "Warning"
}
},
"WriteTo": [
{ "Name": "Console" },
{
"Name": "File",
"Args": {
"path": "./logs/Apiserver.log",
"rollingInterval": "Day"
}
}
]
},
5. Add MediatR
개발자 프롬프트에서 프로젝트 폴더로 이동
(현재 .sln 파일이 있는 폴더라면 .csproj 파일이 있는 폴더로 이동)
아래 명령 실행
dotnet add package MediatR.Extensions.Microsoft.DependencyInjection
Helper 폴더 추가 --> AssemblyHelper.cs 추가
using System.Diagnostics;
using System.Reflection;
using System.Runtime.Loader;
namespace WebApiBasicTutorial.Helper
{
public class AssemblyHelper
{
public static List<Assembly> GetAllAssemblies(SearchOption searchOption = SearchOption.TopDirectoryOnly)
{
List<Assembly> assemblies = new List<Assembly>();
foreach (string assemblyPath in Directory.GetFiles(AppDomain.CurrentDomain.BaseDirectory, "*.dll", searchOption))
{
try
{
var assembly = AssemblyLoadContext.Default.LoadFromAssemblyPath(assemblyPath);
if (assemblies.Find(a => a == assembly) != null)
continue;
assemblies.Add(assembly);
}
catch (Exception ex)
{
Debug.WriteLine(ex.ToString());
}
}
return assemblies;
}
}
}
Program.cs 에 다음 코드 추가
using MediatR;
using WebApiBasicTutorial.Helper;
...
builder.Services..AddMediatR(AssemblyHelper.GetAllAssemblies().ToArray());
6. Add EntityFramework Core
1. mssql 에 대한 DB 공급자 설치
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
2. EF 관련도구 설치
dotnet tool install --global dotnet-ef
** 업데이트가 필요하다면 dotnet tool update 명령을 사용한다. **
dotnet tool update --global dotnet-ef
3. EF design 패키지 설치
dotnet add package Microsoft.EntityFrameworkCore.Design
Infrastructure folder 추가 --> MyDbContext.cs 추가
public class MyDbContext:DbContext
{
public MyDbContext()
{
}
protected MyDbContext(DbContextOptions options) : base(options)
{
}
public MyDbContext(DbContextOptions<MyDbContext> options)
: base(options)
{
}
public virtual DbSet<User> Users { get; set; }
}
Models folder 추가 --> User.cs 추가
public class User
{
public string Id { get; set; } = string.Empty;
public string Name { get; set; } = string.Empty;
public string Email { get; set; } = string.Empty;
}
DbSchema mapping
MyDbContext.cs 에 다음 추가
protected override void OnModelCreating(ModelBuilder modelBuilder)
{
modelBuilder.HasAnnotation("Relational:Collation", "SQL_Latin1_General_CP1_CI_AS");
modelBuilder.Entity<User>(entity =>
{
// Table 이름은 User 로 한다.
entity.ToTable("User");
// Primary key 는 Id 이다.
entity.HasKey(e => e.Id);
// Id 는 필수 값이다.
// Id 는 varchar 형태로 20 length 이다.
entity.Property(e => e.Id)
.IsRequired()
.IsUnicode(false)
.HasMaxLength(20);
// Name 은 256 length 를 갖는 nvarchar 형태이다.
entity.Property(e => e.Name)
.HasMaxLength(256);
// Email 은 256 length 를 갖는 nvarchar 형태이다.
entity.Property(e => e.Email)
.HasMaxLength(256);
});
}
Program.cs 에 다음 코드 추가
using Microsoft.EntityFrameworkCore;
...
var connectionString = builder.Configuration.GetConnectionString("DbServer");
builder.Services.AddDbContext<MyDbContext>(options => options.UseSqlServer(connectionString));
appsettings.json 에 다음 추가
"ConnectionStrings": {
"DbServer": "Server=(localdb)\\mssqllocaldb;Database=WebApiBasicTutorial;Trusted_Connection=True;"
}
7. Add Migration
개발자 명령 프롬프트 실행
개발자 프롬프트에서 솔루션 폴더로 이동
(현재 .csproj 파일이 있는 폴더라면 .sln 파일이 있는 폴더로 이동)
migration
dotnet ef migrations add InitialCreate --context MyDbContext
--output-dir Migrations/MsSqlMigrations
--project WebApiBasicTutorial
--startup-project WebApiBasicTutorial
8. Apply Database
개발자 명령 프롬프트 실행
개발자 프롬프트에서 솔루션 폴더로 이동
(현재 .csproj 파일이 있는 폴더라면 .sln 파일이 있는 폴더로 이동)
database update
dotnet ef database update --context MyDbContext
--project WebApiBasicTutorial
--startup-project WebApiBasicTutorial
--project 에 project 명 기입
--startup-project 에 appsettings.json 이 존재하는 project 기입 해당 정보를 통해 db 연결
현재 Solution 구조
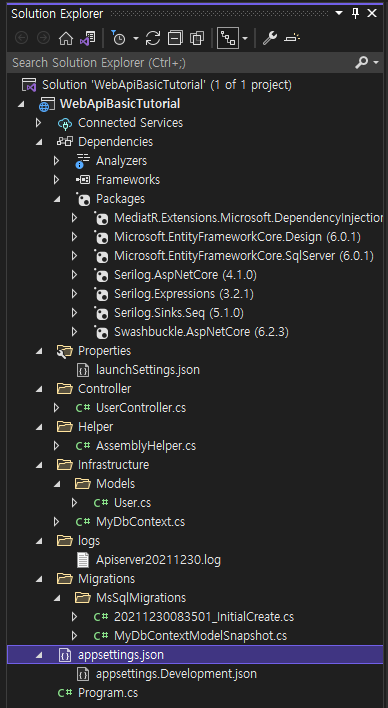
현재 Program.cs 상태
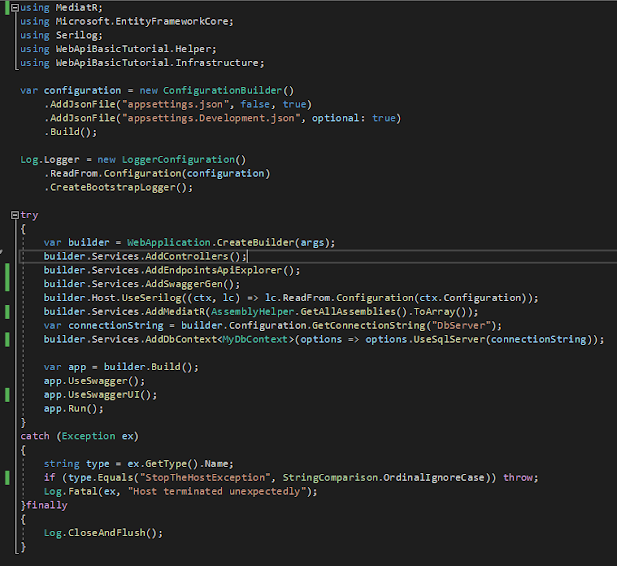
실행
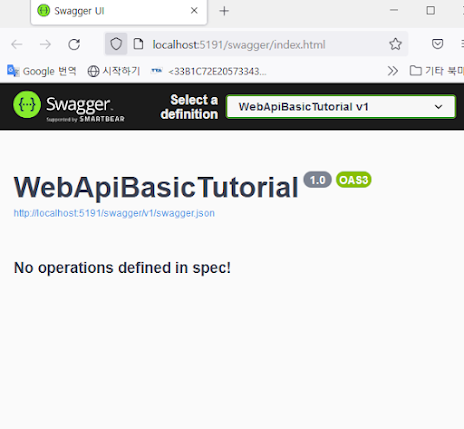
관련동영상
Code
반응형
'ASPNET > WebApi' 카테고리의 다른 글
MySql 에서 schedule 처리 하기 (0) | 2024.04.15 |
---|---|
Quartz 를 이용한 schedule job 처리 (0) | 2024.04.08 |
ASPNET 6 Web Api Basic Tutorial 2 / 2 (Swagger, SeriLog, MediatR, EntityFrameworkCore, Scrutor) (0) | 2022.01.01 |
Dotnet 6 Dependency Injection (0) | 2022.01.01 |