2022. 8. 3. 00:00ㆍMAUI
Person 이라는 객체가 있다고 하자
public class Person
{
public string Name { get; set; }
public int Age { get; set; }
public string Location { get; set; }
}
person 은 위와 같이 Name, Age, Location 을 가지고 있다.
이것을 CollectionView 를 통해 Xaml 에서 표현 하려고 한다고 하자
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
xmlns:local="clr-namespace:DataTemplates"
x:Class="DataTemplates.WithoutDataTemplatePage">
<StackLayout>
<CollectionView>
<CollectionView.ItemsSource>
<x:Array Type="{x:Type local:Person}">
<local:Person Name="Steve" Age="21" Location="USA" />
<local:Person Name="John" Age="37" Location="USA" />
<local:Person Name="Tom" Age="42" Location="UK" />
<local:Person Name="Lucas" Age="29" Location="Germany" />
<local:Person Name="Tariq" Age="39" Location="UK" />
<local:Person Name="Jane" Age="30" Location="USA" />
</x:Array>
</CollectionView.ItemsSource>
</CollectionView>
</StackLayout>
</ContentPage>
위와 같은 식으로 만들 텐데 실제 개체를 표시할때 Person 의 ToString 을 표시한다.
그러므로 아래와 같은 형태가 나온다.
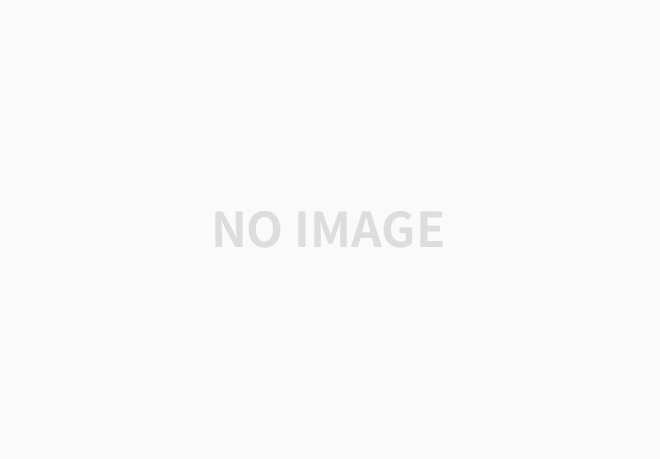
이것은 우리가 의도한 바가 아니다.
그럼 Person 개체의 ToString 을 재정의 해보자
public class Person
{
...
public override string ToString ()
{
return Name;
}
}
이제 다음과 같이 표시된다.
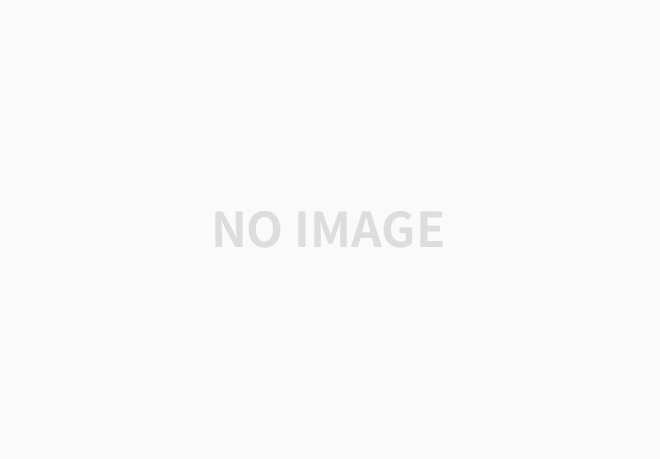
그러나 이 방법은 각 데이터 항목의 모양에 대한 제한된 제어만 제공한다.
유연성을 높이려면 데이터의 모양을 정의하는 DataTemplate을 만들어야 한다.
인라인 DataTemplate 만들기
...
<CollectionView.ItemTemplate>
<DataTemplate>
<Grid ColumnDefinitions="*,*,*" Padding="10,0,10,0">
<Label Text="{Binding Name}" FontAttributes="Bold" />
<Label Grid.Column="1" Text="{Binding Age}" />
<Label Grid.Column="2" Text="{Binding Location}" HorizontalTextAlignment="End" />
</Grid>
</DataTemplate>
</CollectionView.ItemTemplate>
...
형식을 사용하여 DataTemplate 만들기
<?xml version="1.0" encoding="utf-8" ?>
<ContentView xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp1.DataTemplate.PersonView">
<Grid ColumnDefinitions="*,*,*" Padding="10,0,10,0">
<Label Text="{Binding Name}" FontAttributes="Bold" />
<Label Grid.Column="1" Text="{Binding Age}" />
<Label Grid.Column="2" Text="{Binding Location}" HorizontalTextAlignment="End" />
</Grid>
</ContentView>
ContenView 를 상속한 사용자 지정 컨트롤을 만들어서 Grid Layout 을 구성하고
Page 에서 다음과 같이 지정 컨트롤을 사용 한다.
<CollectionView.ItemTemplate>
<DataTemplate>
<local:PersonView />
</DataTemplate>
</CollectionView.ItemTemplate>
DataTemplate을 리소스로 만들기
ContentPage.Resources 를 이용하여 DataTemplate 을 구성하고
CollectionView 의 ItemTemplate 속성에 StaticResource 를 통해 DataTemplate 을 연결 할 수 있다.
<ContentPage.Resources>
<ResourceDictionary>
<DataTemplate x:Key="personTemplate">
<Grid ColumnDefinitions="*,*,*" Padding="10,0,10,0">
<Label Text="{Binding Name}" FontAttributes="Bold" />
<Label Grid.Column="1" Text="{Binding Age}" />
<Label Grid.Column="2" Text="{Binding Location}" HorizontalTextAlignment="End" />
</Grid>
</DataTemplate>
</ResourceDictionary>
</ContentPage.Resources>
<CollectionView ItemTemplate="{StaticResource personTemplate}">
<CollectionView.ItemsSource>
<x:Array Type="{x:Type local:Person}">
<local:Person Name="Steve" Age="21" Location="USA" />
<local:Person Name="John" Age="37" Location="USA" />
<local:Person Name="Tom" Age="42" Location="UK" />
<local:Person Name="Lucas" Age="29" Location="Germany" />
<local:Person Name="Tariq" Age="39" Location="UK" />
<local:Person Name="Jane" Age="30" Location="USA" />
</x:Array>
</CollectionView.ItemsSource>
</CollectionView>
DataTemplateSelector 만들기
여러가지 모양의 DataTemplate 을 만들어서 그중 하나를 선택 하는데 사용된다.
런타임에 모양을 선택할 수 있다.
데이터 템플릿 선택기는 DataTemplateSelector에서 상속되는 클래스를 만들어 구현한다.
그런 다음, 메서드를 OnSelectTemplate 재정의하여 특정 DataTemplate한 다음을 반환해야한다.
<DataTemplate x:Key="validPersonTemplate">
<Grid ColumnDefinitions="*,*,*" Padding="10,0,10,0">
<Label Text="{Binding Name}" FontAttributes="Bold" TextColor="Blue" />
<Label Grid.Column="1" Text="{Binding Age}" TextColor="Blue" />
<Label Grid.Column="2" Text="{Binding Location}" HorizontalTextAlignment="End" TextColor="Blue" />
</Grid>
</DataTemplate>
<DataTemplate x:Key="invalidPersonTemplate">
<Grid ColumnDefinitions="*,*,*" Padding="10,0,10,0">
<Label Text="{Binding Name}" FontAttributes="Bold" TextColor="Red" />
<Label Grid.Column="1" Text="{Binding Age}" TextColor="Red"/>
<Label Grid.Column="2" Text="{Binding Location}" HorizontalTextAlignment="End" TextColor="Red"/>
</Grid>
</DataTemplate>
<local:PersonDataTemplateSelector x:Key="personDataTemplateSelector"
ValidTemplate="{StaticResource validPersonTemplate}"
InvalidTemplate="{StaticResource invalidPersonTemplate}" />
<StackLayout>
<CollectionView x:Name="collectionView"
ItemTemplate="{StaticResource personDataTemplateSelector}" >
<CollectionView.ItemsSource>
<x:Array Type="{x:Type local:Person}">
<local:Person Name="Steve" Age="15" Location="USA" />
<local:Person Name="John" Age="37" Location="USA" />
<local:Person Name="Tom" Age="8" Location="UK" />
<local:Person Name="Lucas" Age="29" Location="Germany" />
<local:Person Name="Tariq" Age="39" Location="UK" />
<local:Person Name="Jane" Age="11" Location="USA" />
</x:Array>
</CollectionView.ItemsSource>
</CollectionView>
</StackLayout>
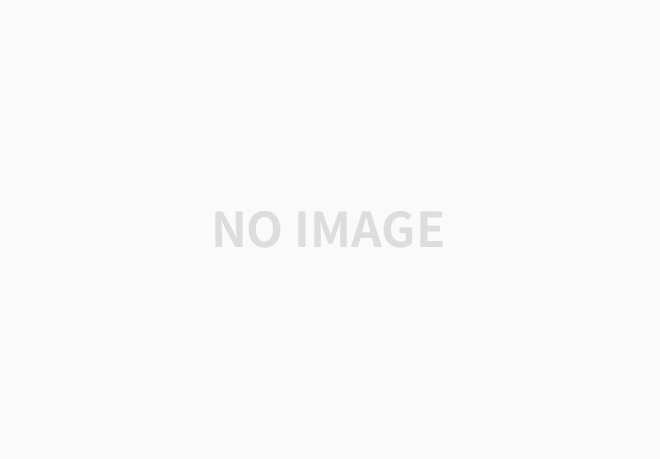
관련영상
'MAUI' 카테고리의 다른 글
.NET MAUI - Triggers (2) (0) | 2022.08.05 |
---|---|
.NET MAUI - Triggers (1) (0) | 2022.08.04 |
.NET MAUI - Control Templates (0) | 2022.08.02 |
.NET MAUI - Binding Command (0) | 2022.08.01 |
.NET MAUI - Binding Relative bindings (0) | 2022.07.29 |