2022. 8. 8. 00:00ㆍMAUI
.NET 다중 플랫폼 앱 UI(.NET MAUI) Behavior 를 사용하면 서브클래스하지 않고도 사용자 인터페이스 컨트롤에 기능을 추가할 수 있다. 기능은 Behavior 에서 구현되고 컨트롤 자체의 일부였던 것처럼 컨트롤에 연결된다.
Behavior을 사용하면 코드가 컨트롤에 간결하게 첨부되고 컨트롤의 API와 직접 상호 작용하기 때문에, 대개 코드 숨김으로 작성해야 하는 코드를 대체한다.
.NET MAUI는 두 가지 유형의 Behavior 을 지원한다.
- Attached Behavior은 Attached Property가 하나 이상 있는 static 클래스이다.
- .NET MAUI Behavior 은 Behavior<T> 클래스에서 파생되는 클래스 T 이다.
Attached Behavior
Attached Property 가 하나 이상 있는 static class . attached property 는 특수한 속성의 바인딩 가능 속성
XAML 에서 마침표로 구분된 클래스 및 Property 이름을 포함 하는 특성으로 인식할 수 있다.
Attached Property
속성 값이 변경되면 실행될 propertyChanged 대리자를 정의할 수 있다.
propertyChanged 대리자는 이를 연결할 컨트롤의 속성에 대한 이전 및 새 값을 전달 한다.
Entry 에 Text 를 입력할때 숫자가 아닐경우 빨강색으로 Text Color 를 만드는 Behavior 를 만들어보자
AttachedNumericValidationBehavior.cs
namespace MauiApp1.Behaviors;
public static class AttachedNumericValidationBehavior
{
public static readonly BindableProperty AttachBehaviorProperty =
BindableProperty.CreateAttached("AttachBehavior", typeof(bool), typeof(AttachedNumericValidationBehavior), false, propertyChanged: OnAttachBehaviorChanged);
public static bool GetAttachBehavior(BindableObject view)
{
return (bool)view.GetValue(AttachBehaviorProperty);
}
public static void SetAttachBehavior(BindableObject view, bool value)
{
view.SetValue(AttachBehaviorProperty, value);
}
static void OnAttachBehaviorChanged(BindableObject view, object oldValue, object newValue)
{
Entry entry = view as Entry;
if (entry == null)
{
return;
}
bool attachBehavior = (bool)newValue;
if (attachBehavior)
{
entry.TextChanged += OnEntryTextChanged;
}
else
{
entry.TextChanged -= OnEntryTextChanged;
}
}
static void OnEntryTextChanged(object sender, TextChangedEventArgs args)
{
double result;
bool isValid = double.TryParse(args.NewTextValue, out result);
((Entry)sender).TextColor = isValid ? Colors.Black : Colors.Red;
}
}
AttachedNumericValidationPage.xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp1.Behaviors.AttachedNumericValidationPage"
xmlns:local="clr-namespace:MauiApp1.Behaviors"
Title="AttachedNumericValidationPage">
<StackLayout Padding="10,60,10,0">
<Label Text="Red when the number isn't valid"
FontSize="12" />
<Entry Placeholder="Enter a System.Double"
local:AttachedNumericValidationBehavior.AttachBehavior="true" />
</StackLayout>
</ContentPage>
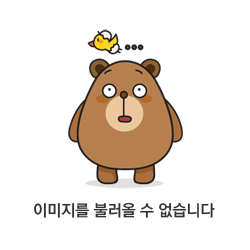
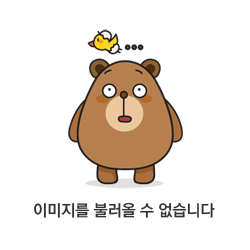
또한 Style 형태로 사용 할 수 도 있다.
<Style x:Key="NumericValidationStyle" TargetType="Entry">
<Style.Setters>
<Setter Property="local:NumericValidationStyleBehavior.AttachBehavior" Value="true" />
</Style.Setters>
</Style>
<Entry Placeholder="Enter a System.Double" Style="{StaticResource NumericValidationStyle}">
동작 제거는 다음과 같이 한다.
Behavior toRemove = entry.Behaviors.FirstOrDefault(b => b is NumericValidationStyleBehavior);
if (toRemove != null)
{
entry.Behaviors.Remove(toRemove);
}
entry.Behaviors.Clear();
.NET MAUI behaviors
Behavior 또는 Behavior<T> 클래스에서 파생되어 생성된다.
- Behavior 또는 Behavior<T> 클래스에서 상속하는 클래스를 만든다. 여기서 T는 컨트롤 형식이다.
- 필요한 설정을 수행하려면 OnAttachedTo 메서드를 재정의한다.
- 필요한 정리를 수행하려면 OnDetachingFrom 메서드를 재정의한다.
- Behavior 의 핵심 기능을 구현한다.
namespace MauiAppTest.Behaviors;
public class NumericValidationBehavior : Behavior<Entry>
{
protected override void OnAttachedTo(Entry entry)
{
entry.TextChanged += OnEntryTextChanged;
base.OnAttachedTo(entry);
// Perform setup
}
protected override void OnDetachingFrom(Entry entry)
{
entry.TextChanged -= OnEntryTextChanged;
base.OnDetachingFrom(entry);
// Perform clean up
}
void OnEntryTextChanged(object sender, TextChangedEventArgs args)
{
double result;
bool isValid = double.TryParse(args.NewTextValue, out result);
((Entry)sender).TextColor = isValid ? Colors.Black : Colors.Red;
}
}
이밖에도 Behavior<T> 를 이용해서 Style 에 등록 하는 방법이 있으나 개인적으로는 별로 추천하고 싶지 않다.
(복잡하고 중복작업을 하는 이상한 방식이다. 이런 방식은 그냥 억지스럽다는 생각밖에 들지 않는다.)
만약 꼭 Style 에 등록 하고 싶다면 이전에 사용했던 Attached Behavior 를 이용하자.
관련영상
'MAUI' 카테고리의 다른 글
.NET MAUI - MVVM ObservableObject (0) | 2022.08.10 |
---|---|
.NET MAUI - MVVM and CommunityToolkit.Mvvm (0) | 2022.08.09 |
.NET MAUI - Triggers (2) (0) | 2022.08.05 |
.NET MAUI - Triggers (1) (0) | 2022.08.04 |
.NET MAUI - Data Templates (0) | 2022.08.03 |