2023. 5. 29. 00:00ㆍPython/FASTAPI
*이 강좌는 Python, Poetry, FASTAPI 에 대한 기본적인 지식이 있다고 가정합니다.*
poetry 를 이용하여 project 를 만들어 보자
poetry new fastapi_vertical_slice
이제 해당 폴더로 이동하자 그리고 shell 을 통해 격리환경으로 들어가자
poetry shell
이제 fastapi 를 설치하자 dotenv 와 uvicorn 등을 사용할 테니 all 로 설치하자
poetry add fastapi[all]
이제 visual studio code (또는 다른 editor) 로 해당 folder 를 열자
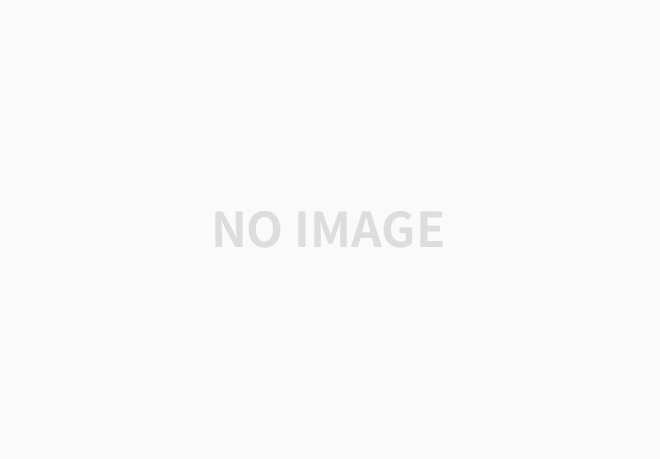
위와 같이 구성되어 있을 것이다.
일단 folder 의 이름을 바꿀 것이다.
fastapi_vertical_slice --> src 로 변경
그리고 src 밑에 main.py 를 추가하자
main.py
from fastapi import FastAPI
app = FastAPI(title="FastAPI, vertical slice architecture")
@app.get("/")
def read_root():
return {"hello": "world"}
@app.post("/")
def read_root():
return {"hello": "world"}
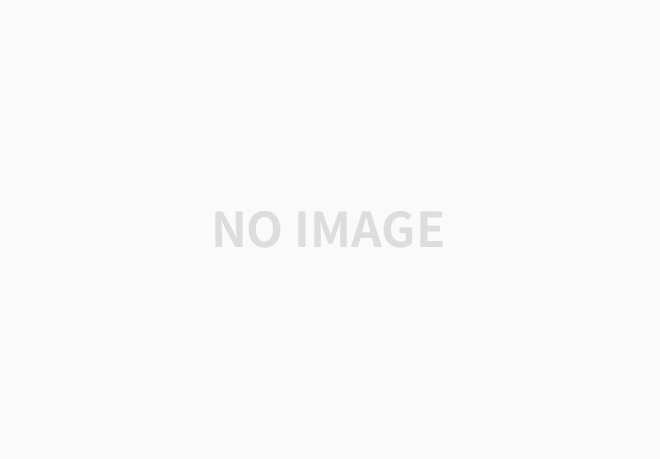
이제 이 fastapi app 을 실행해보자
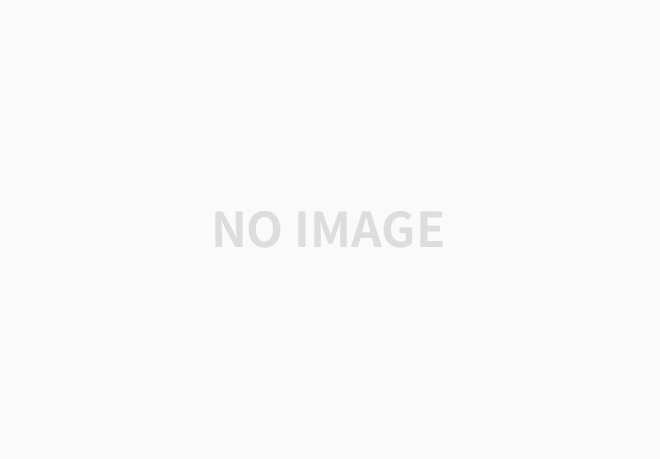
위와 같이 실행되면 정상 실행된 것이다.
이제 구성을 조금 바꿔 볼 것이다.
Vertical Slice Architecture 라고 부르는 형태로 구성을 할 것이다.
Vertical Slice Architecture 자세한 내용은 아래를 참조하자
https://jimmybogard.com/vertical-slice-architecture/
Vertical Slice Architecture
Many years back, we started on a new, long term project, and to start off with, we built the architecture around an onion architecture. Within a couple of months, the cracks started to show around this style and we moved away from that architecture and tow
jimmybogard.com
그래서 이번에는 일단 folder 구조를 조정하고 main.py 에 집중되어 있는 로직을 분산할 것이다.
*__pycache__ 형태의 폴더가 생성되어 있는 것이 보일텐데 visual studio code 에서 exclude 해버리자*
file --> preferences --> settings 이동 (ctrl + ,)
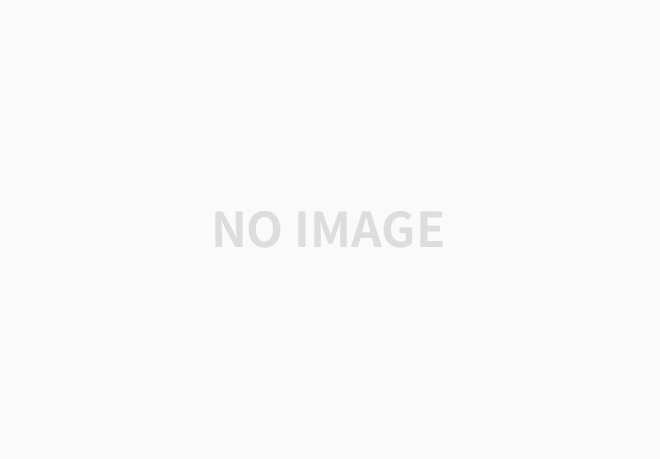
Workspace --> Files: Exclude --> Add Pattern
**/__pycache__
이렇게 해서 __pycache__ 는 Project 에서 안나오게 하자 (다른 editor 들도 관련 기능이 있을테니 찾아보자)
그리고 project 구조를 다음과 같이 구성하자
features, infrastructure, shared 라는 folder 를 추가했다.
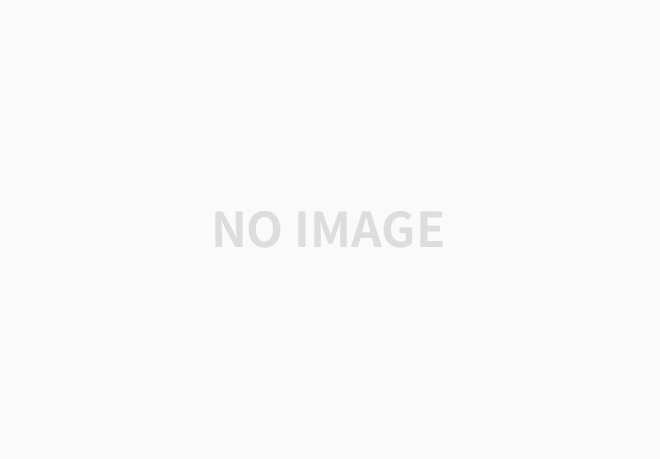
* 작업중에 import "fastapi" could not be resolved 가 계속 나올경우 *
1) Press Ctrl + Shift + P on your VsCode
2) Python : Select Interpreter
3) 우리는 poetry 용가상환경에 들어와 있으니
poetry 용 가상환경 interpreter 를 설정하자 (python version 을 맞추는것)
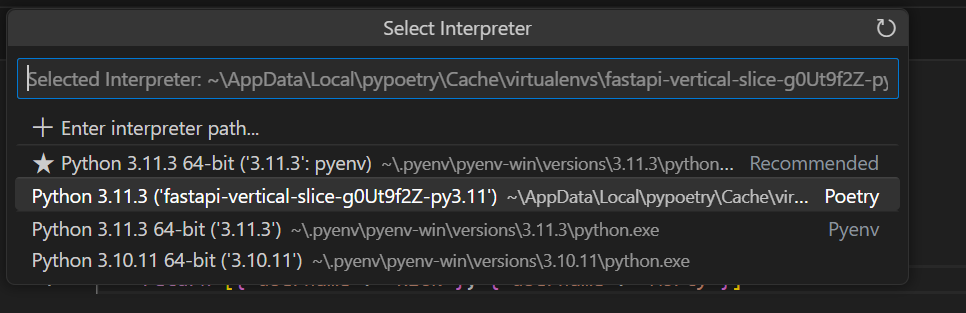
추가
features --> hello --> endpoints.py
endpoints.py
from fastapi import APIRouter
router = APIRouter()
@router.get("/", tags=["hello"])
def read_root():
return {"hello": "world"}
@router.post("/", tags=["hello"])
def read_root():
return {"hello": "world"}
main.py
from fastapi import FastAPI
from .features.hello import endpoints
app = FastAPI(title="FastAPI, vertical slice architecture")
app.include_router(endpoints.router)
이제 uvicorn src.main:app --reload 를 통해 실행해보자
아마 정상적으로 실행될 것이다.
main.py 에 있는 내용을 features/hello/endpoints.py 로 옮겼다.
그리고 main.py 에서 router 를 include 하였다.
여기만 보면 크게 달라진 점은 없어 보인다.
이제 mediator 를 이용하여 event 를 통해 처리 하는 방식으로 변경해 보겠다.
mediator 관련 library 를 설치하자
poetry add mediatr
이제 features-->hello 밑에 get.py 와 post.py 를 생성하자
get.py
from mediatr import Mediator
class GetHello():
pass
@Mediator.handler
async def handle(request: GetHello):
return {"hello": "world(Get)"}
post.py
from mediatr import Mediator
class PostHello():
pass
@Mediator.handler
async def handle(request: PostHello):
return {"hello": "world (Post)"}
endpoints.py 는 다음과 같이 변경한다.
endpoints.py
from fastapi import APIRouter
from mediatr import Mediator
from .get import GetHello
from .post import PostHello
router = APIRouter()
@router.get("/", tags=["hello"])
async def read_root():
mediator = Mediator()
request = GetHello()
return await mediator.send_async(request)
@router.post("/", tags=["hello"])
async def read_root():
mediator = Mediator()
request = PostHello()
return await mediator.send_async(request)
mediatr 사용법은 다음을 참고하자
https://github.com/megafetis/mediatr_py
GitHub - megafetis/mediatr_py: Mediator implementation for python 3.6+ (version >=1.2<1.3 for python 3.5), that supports pipline
Mediator implementation for python 3.6+ (version >=1.2<1.3 for python 3.5), that supports pipline behaviors, async executing - GitHub - megafetis/mediatr_py: Mediator implementation for pytho...
github.com
아주 극단적인 예제였지만 내용을 살펴보면 다음과 같다.
features 아래 hello 라는 folder 를 만들고
endpoints 를 이용해서 route 관련 처리를 한다.
mediatR 을 이용해서 event 방식으로 handler 에 요청을 보낸다.
hello 의 2 method 인 get 과 post 각각 handler 로 분리 하여 처리 하였다.
get 과 post 에서 domain model 과 data model 그리고 external service 들을 조합하여 기능을 구현한다.
이렇게 get, post 가 hello 라는 폴더안에 응집 되어 있고 get 에는 get 에 대한 request class 와 handler 가 모여있다.
hello 라는 folder 를 따로 때어내면 하나의 기능이 구현되어 있다.
이런 방식을 vertical slice architecture 라고 한다.
이 방식의 개발은 빠른 개발을 유도한다. 구현이 최우선 이므로 copy and paste 도 적극 활용해야한다.
중복된 내용들은 향후 refactoring 을 통해 재거한다.
처음부터 layer 를 구분하려 하지 말고 refactoring 을 통해 정말 필요한 것들만 구분하도록 하자
관련영상