2022. 8. 18. 00:00ㆍMAUI
의존성 주입은 하나의 객체가 다른 객체의 의존성을 제공하는 테크닉이다. "의존성"은 예를 들어 서비스로 사용할 수 있는 객체이다. 클라이언트가 어떤 서비스를 사용할 것인지 지정하는 대신, 클라이언트에게 무슨 서비스를 사용할 것인지를 말해주는 것이다.
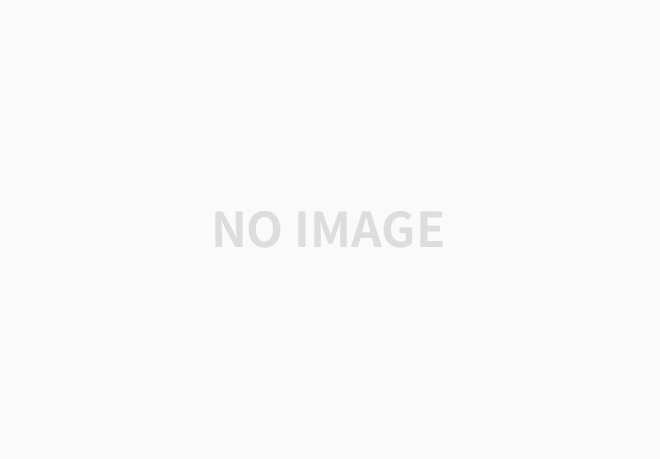
.NET MAUI 에서는 Microsoft.Extensions.DependencyInjection 을 이용하여 DI 를 구현한다.
https://yogingang.tistory.com/2
Dotnet 6 Dependency Injection
종속성 클래스와 해당 종속성 간의 Ioc (Inversion of Control)를 실현하는 기술로 DI (Dependency Injection) 라는 디자인 패턴을 지원한다. 종류 (서비스 수명) Transient : 요청할 때마다 만들어 짐, 요청이..
yogingang.tistory.com
https://docs.microsoft.com/ko-kr/dotnet/core/extensions/dependency-injection
종속성 주입 - .NET
.NET에서 종속성 주입을 구현하는 방법 및 사용 방법을 알아봅니다.
docs.microsoft.com
이와 같은 형태로 MAUI 에서 Dependency Injection 을 이용할 수 있다.
자세한 내용은 위 참조를 확인 하도록 하고 여기서는 MAUI 와 통합해서 사용해 보겠다.
일단 다음과 같이 몇가지 코드를 작성한다.
HelloWorldClass.cs
namespace MauiApp1.MVVM.DependencyInjection;
public interface IHelloWorldClass
{
string Execute();
}
public class HelloWorldClass : IHelloWorldClass
{
public string Execute() => $"{DateTime.Now} Hello World!";
}
IncrementCounterPage.xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp1.MVVM.DependencyInjection.IncrementCounterPage"
xmlns:local="clr-namespace:MauiApp1.MVVM.DependencyInjection"
Title="IncrementCounterPage">
<VerticalStackLayout>
<Label Text="{Binding Counter, Mode=OneWay}"/>
<Button
Text="Click me!"
Command="{Binding IncrementCounterCommand}"/>
<Label Text="{Binding Message, Mode=OneWay}"/>
<Button
Text="Change message"
Command="{Binding ChangeMessageCommand}"/>
</VerticalStackLayout>
</ContentPage>
IncrementCounterPage.xaml.cs
namespace MauiApp1.MVVM.DependencyInjection;
public partial class IncrementCounterPage : ContentPage
{
public IncrementCounterPage(IncrementCounterViewModel incrementCounterViewModel)
{
InitializeComponent();
BindingContext = incrementCounterViewModel;
}
}
IncrementCounterViewModel.cs
using CommunityToolkit.Mvvm.ComponentModel;
using CommunityToolkit.Mvvm.Input;
namespace MauiApp1.MVVM.DependencyInjection;
public partial class IncrementCounterViewModel : ObservableObject
{
public IncrementCounterViewModel(IHelloWorldClass helloWorld)
{
_helloWorld = helloWorld;
}
[ObservableProperty]
private int _counter;
[ObservableProperty]
private string _message;
private readonly IHelloWorldClass _helloWorld;
[RelayCommand]
private void IncrementCounter() => Counter++;
[RelayCommand]
private void ChangeMessage()
{
Message = _helloWorld.Execute();
}
}
자 대부분의 코드를 확인해서 Constructor (생성자) 를 보면
Constructor Injection 을 통해 각각의 class 들을 주입 받을걸 확인 해 볼수 있다.
IncrementCounterPage 는 IncrementCounterViewModel 을 주입받았고
IncrementCounterViewModel 은 IHelloWorldClass 를 주입받았다.
자 그럼 위 class 들을 어디서 register 했을까?
MauiProgram.cs 로 이동하자
MauiProgram.cs
using MauiApp1.MVVM.DependencyInjection;
namespace MauiApp1;
public static class MauiProgram
{
public static MauiApp CreateMauiApp()
{
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold");
});
builder.Services.AddTransient<IHelloWorldClass, HelloWorldClass>();
builder.Services.AddTransient<IncrementCounterViewModel>();
builder.Services.AddTransient<IncrementCounterPage>();
return builder.Build();
}
}
아래 builder.Services.AddXXXX 를 통해 register 하고 있다.
App --> Shell --> Page 순서로 진행하게 되는데 이때 Page 부터는 Services 에 등록해야 한다.
builder.Services.AddTransient<IHelloWorldClass, HelloWorldClass>();
builder.Services.AddTransient<IncrementCounterViewModel>();
builder.Services.AddTransient<IncrementCounterPage>();
위와 같이 코딩하게 되면 각 class 들의 instance 를 DotNet Dependency Injection System 을 통해 관리 하게 된다.
실행화면
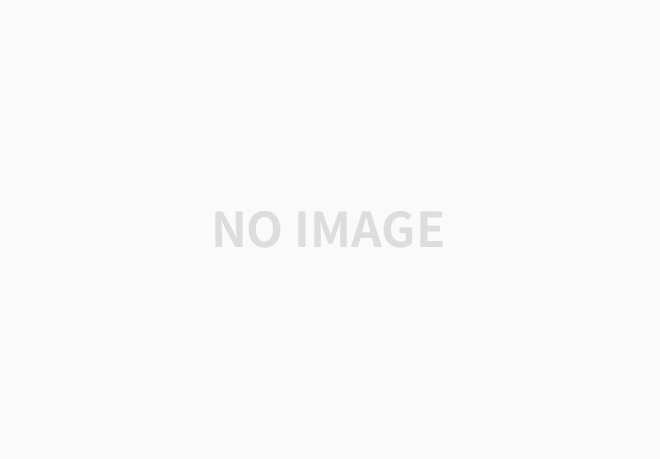
관련영상
'MAUI' 카테고리의 다른 글
.NET MAUI - Xaml Compilation (for HighPerformance) (0) | 2022.08.22 |
---|---|
.NET MAUI - MVVM Dependency Injection 2/2 (AutoScan with Scrutor) (0) | 2022.08.19 |
.NET MAUI - MVVM SourceGenerator (0) | 2022.08.17 |
.NET MAUI - MVVM Messenger (0) | 2022.08.16 |
.NET MAUI - MVVM Command (0) | 2022.08.15 |