2022. 8. 23. 00:00ㆍMAUI
.NET MAUI 커뮤니티 도구 키트는 애니메이션, 동작, 변환기, 효과 및 도우미를 포함하여 MAUI를 사용하여 애플리케이션 개발을 위한 재사용 가능한 요소 모음이다. NET MAUI를 사용하여 iOS, Android, macOS 및 WinUI 애플리케이션을 빌드할 때 일반적인 개발자 작업을 간소화하고 보여 준다.
MAUI 커뮤니티 도구 키트는 신규 또는 기존 프로젝트에 NuGet 패키지를 통해 설치하여 사용할 수 있다.
설치하기
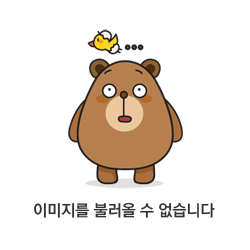
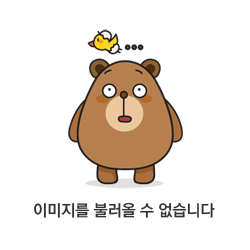
MauiProgram.cs 로 이동하여 다음 코드를 추가하자
...
using CommunityToolkit.Maui;
...
...
// UseMauiCommunityToolkit() 추가
var builder = MauiApp.CreateBuilder();
builder
.UseMauiApp<App>()
.UseMauiCommunityToolkit()
.ConfigureFonts(fonts =>
{
fonts.AddFont("OpenSans-Regular.ttf", "OpenSansRegular");
fonts.AddFont("OpenSans-Semibold.ttf", "OpenSansSemibold");
});
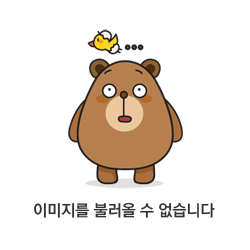
설치및 설정이 완료되었다면 다음을 확인해 보자
기본적으로 CommunityToolkit.Mvvm (8.0) 또한 설치되어 있다고 가정하고 설명합니다.
Dependency Injection 와 Cusomization 을 활용하여 Page,ViewModel, ServiceClass 들을 자동 등록중입니다.
https://yogingang.tistory.com/337
xaml 상단에 다음과 같은 namespace 를 포함해야 한다고 Manual 에 되어 있으나
Snackbar 와 Toast 에 대해서는 크게 영향을 주지 않는것 같다.
(Xaml 을 직접 수정하지 않아서 그런것으로 보인다. behind code 에서 모두 설정)
xmlns:toolkit="http://schemas.microsoft.com/dotnet/2022/maui/toolkit"
Alerts
Alerts 는 사용자에게 정보를 알리는 방법을 제공한다.
Snackbar 와 Toast 라는 두가지 종류로 구성되어 있다.
Snackbar
화면 아래쪽에 표시되는 시간이 제한된 경고. 일정 시간이 지나면 사라진다.
모든 IView 를 상속한 view 에 고정할 수 있다.
아래와 같이 직접 만들어 전달 할 수 있다.
var snackbar = Snackbar.Make(text, action, actionButtonText, duration, snackbarOptions);
await snackbar.Show(cancellationTokenSource.Token);
또는 확장 메서드를 이용하는 방법이 있다. (VisualElement 를 상속받은 형태이거나 VisualElement type 이어야한다.)
await MyVisualElement.DisplaySnackbar("Snackbar is awesome. It is anchored to MyVisualElement");
예제)
SnackbarPage.xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp1.Alerts.SnackbarPage"
xmlns:local ="clr-namespace:MauiApp1.Alerts"
x:DataType="local:SnackbarViewModel"
Title="SnackbarPage">
<ContentPage.Resources>
<Style TargetType="Label">
<Setter Property="FontSize" Value="24" />
<Setter Property="TextColor" Value="Blue" />
</Style>
</ContentPage.Resources>
<VerticalStackLayout Spacing="10">
<Label Text="{Binding Counter, Mode=OneWay}"/>
<Button
Text="Click me!"
Command="{Binding IncrementCounterCommand}" Clicked="OnClickedSnackbar"/>
<Label Text="{Binding Message, Mode=OneWay}"
TextColor="Red"/>
<Button
Text="Change message"
Command="{Binding ChangeMessageCommand}" Clicked="OnClickedCustomizeSnackbar"/>
</VerticalStackLayout>
</ContentPage>
SnackbarPage.xaml.cs
using CommunityToolkit.Maui.Alerts;
using CommunityToolkit.Maui.Core;
using MauiApp1.InjectableServices;
using Font = Microsoft.Maui.Font;
namespace MauiApp1.Alerts;
public partial class SnackbarPage : ContentPage, ITransientService
{
public SnackbarPage(SnackbarViewModel snackbarViewModel)
{
InitializeComponent();
//BindingContext = new SnackbarViewModel(new HelloWorldClass());
BindingContext = snackbarViewModel;
}
private async void OnClickedSnackbar(object sender, EventArgs e)
{
await this.DisplaySnackbar("SnackBar");
}
private async void OnClickedCustomizeSnackbar(object sender, EventArgs e)
{
CancellationTokenSource cancellationTokenSource = new CancellationTokenSource();
var snackbarOptions = new SnackbarOptions
{
BackgroundColor = Colors.Red,
TextColor = Colors.Green,
ActionButtonTextColor = Colors.Blue,
CornerRadius = new CornerRadius(10),
Font = Font.SystemFontOfSize(14),
ActionButtonFont = Font.SystemFontOfSize(14),
CharacterSpacing = 0.5
};
var snackbar = Snackbar.Make(
"This is a Snackbar",
async () => await DisplayAlert("Snackbar ActionButton Tapped", "The user has tapped the Snackbar ActionButton", "OK"),
"Close",
TimeSpan.FromSeconds(3),
snackbarOptions);
await snackbar.Show(cancellationTokenSource.Token);
}
}
SnackbarViewModel.cs
using CommunityToolkit.Mvvm.ComponentModel;
using CommunityToolkit.Mvvm.Input;
using MauiApp1.InjectableServices;
namespace MauiApp1.Alerts;
public partial class SnackbarViewModel : ObservableObject, ITransientService
{
public SnackbarViewModel(IHelloWorldClass helloWorld)
{
_helloWorld = helloWorld;
}
[ObservableProperty]
private int _counter;
[ObservableProperty]
private string _message;
private readonly IHelloWorldClass _helloWorld;
[RelayCommand]
private void IncrementCounter() => Counter++;
[RelayCommand]
private void ChangeMessage()
{
Message = _helloWorld.Execute();
}
}
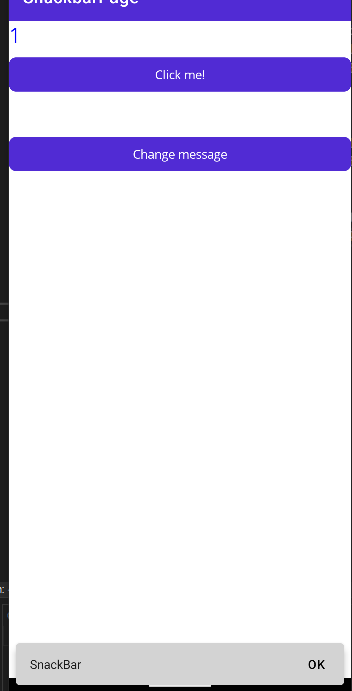
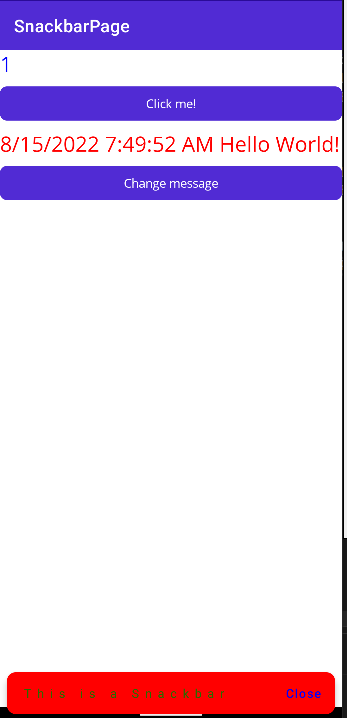
Toast
화면 아래쪽에 표시되는 시간이 제한된 경고. 일정 시간이 지나면 사라진다.
var toast = Toast.Make("This is a default Toast.");
await toast.Show();
var toast = Toast.Make("This is a big Toast.", ToastDuration.Long, 30d);
await toast.Show();
ToastPage.xaml
<?xml version="1.0" encoding="utf-8" ?>
<ContentPage xmlns="http://schemas.microsoft.com/dotnet/2021/maui"
xmlns:x="http://schemas.microsoft.com/winfx/2009/xaml"
x:Class="MauiApp1.Alerts.ToastPage"
xmlns:local ="clr-namespace:MauiApp1.Alerts"
x:DataType="local:SnackbarViewModel"
Title="ToastPage">
<ContentPage.Resources>
<Style TargetType="Label">
<Setter Property="FontSize" Value="24" />
<Setter Property="TextColor" Value="Blue" />
</Style>
</ContentPage.Resources>
<VerticalStackLayout Spacing="10">
<Label Text="{Binding Counter, Mode=OneWay}"/>
<Button
Text="Click me!"
Command="{Binding IncrementCounterCommand}" Clicked="OnClickedToast"/>
<Label Text="{Binding Message, Mode=OneWay}"
TextColor="Red"/>
<Button
Text="Change message"
Command="{Binding ChangeMessageCommand}" Clicked="OnClickedCustomizeToast"/>
</VerticalStackLayout>
</ContentPage>
ToastPage.xaml.cs
using CommunityToolkit.Maui.Alerts;
using CommunityToolkit.Maui.Core;
using MauiApp1.InjectableServices;
using Font = Microsoft.Maui.Font;
namespace MauiApp1.Alerts;
public partial class ToastPage : ContentPage, ITransientService
{
public ToastPage(SnackbarViewModel snackbarViewModel)
{
InitializeComponent();
//BindingContext = new SnackbarViewModel(new HelloWorldClass());
BindingContext = snackbarViewModel;
}
private async void OnClickedToast(object sender, EventArgs e)
{
var toast = Toast.Make("Toast");
await toast.Show();
}
private async void OnClickedCustomizeToast(object sender, EventArgs e)
{
var toast = Toast.Make("Big Toast.", ToastDuration.Long, 30d);
await toast.Show();
}
}
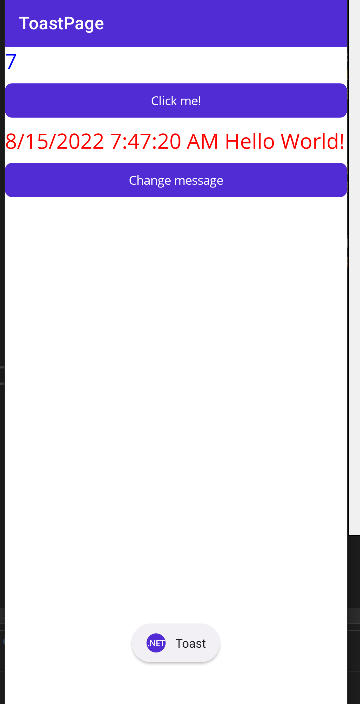
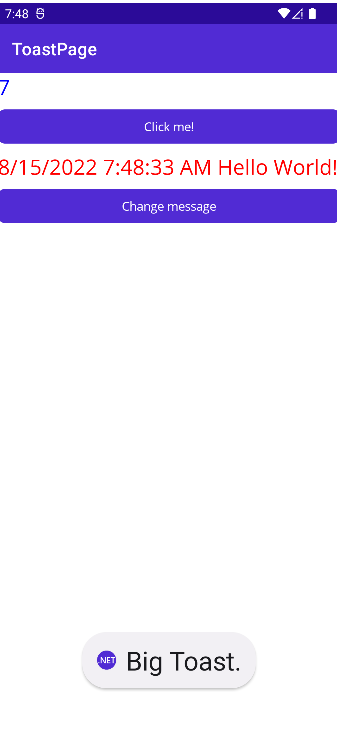
관련영상
'MAUI' 카테고리의 다른 글
.NET MAUI - CommunityToolkit.Maui 유용한 Behavior 2 (0) | 2022.08.25 |
---|---|
.NET MAUI - CommunityToolkit.Maui 유용한 Behavior 1 (0) | 2022.08.24 |
.NET MAUI - Xaml Compilation (for HighPerformance) (0) | 2022.08.22 |
.NET MAUI - MVVM Dependency Injection 2/2 (AutoScan with Scrutor) (0) | 2022.08.19 |
.NET MAUI - MVVM Dependency Injection 1/2 (0) | 2022.08.18 |